In the last article we saw how to mimic the UIKit table view by using the SwiftUI List container.
In this article we will go a step further to make the List expandable with a disclosure indicator that will indicate the state of the cell (expanded vs collapsed).
We will display a list of posts. Each post title will be displayed as a primary content. On click on the cell it will expand to reveal the post full description.
To start off, download the starter project here.
Take some time to explore the project files:
– posts.json contains a collection of data representing the posts that will be displayed in our List.
– PostsViewModel.swift implements the ViewModel which will be called to fetch the data and return it to the view. It also implements the logic to extract and decode the JSON data from the posts file.
If you run the app it won’t show the data yet as we didn’t implement the binding part to populate the data in the List.
Select ContentView.swift and remove the content inside the body‘s computed property. Also, add a declaration for the posts variable at the ContentView struct implementation:
@State var posts: [Post] = []
The posts variable is annotated with the State property wrapper to make our code respond automatically to any change to its value.
Place the following code in the body computed property:
List(posts, id: \.title) { post in DisclosureGroup { Text(post.body) } label: { Text(post.title).font(.title) } } .padding() .onAppear { let viewModel = PostsViewModel() self.posts = viewModel.getPosts() ?? [] }
The List will observe the posts variable value and use it as the data source to populate its content. Then It will loop through the posts array and display each element in a DisclosureGroup – the post.body being the expandable area while the post.title is the always displayed part of the cell.
We also set a padding to the List edges and called the getPosts right before the List is appeared.
Run the project and enjoy the expandable content 🙂
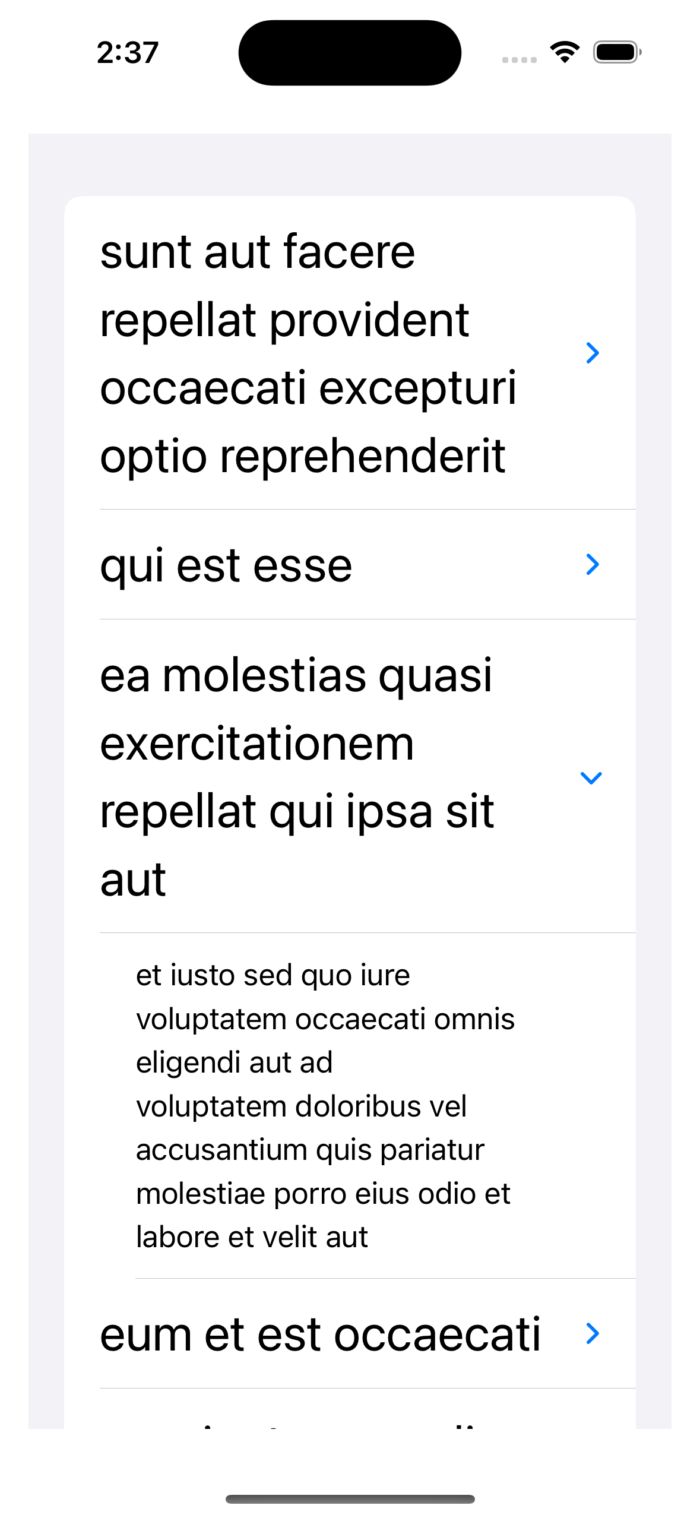
How much code would we need to implement if we would reproduce the same behavior with UIKit? 😅
You can clone the final project code in github.
Catch you in the next article 👋
Thanks!